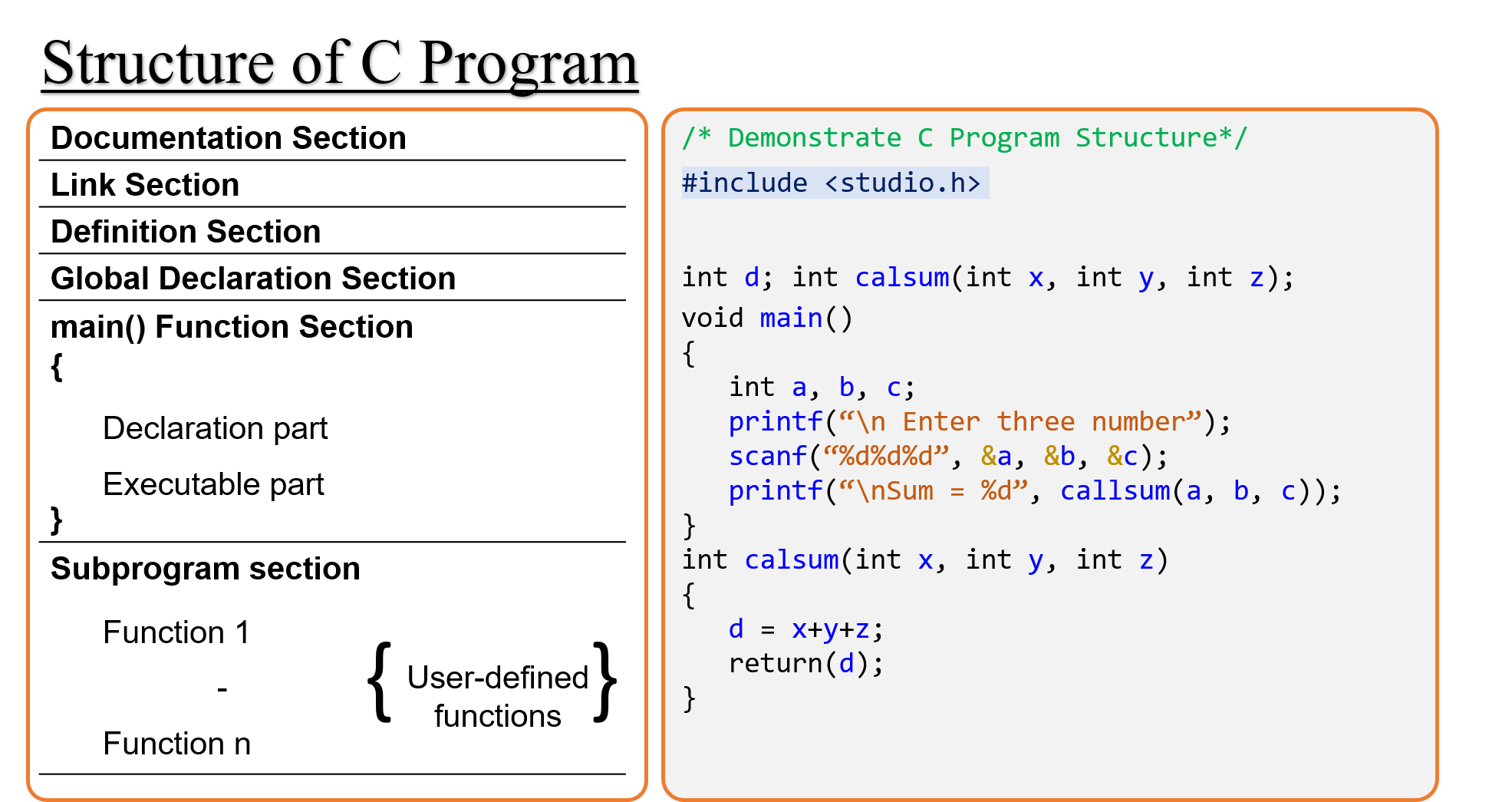
A C program is divided into six sections: Documentation, Link, Definition, Global Declaration, Main() Function, and
Subprograms. While the main function is compulsory, the rest are optional in the structure of the C program.
- Documentation Section
-
- The documentation section is the part of the program where the programmer gives the details associated with the program.
He usually gives the name of the program, the details of the author and other details like the time of coding and description.
It gives anyone reading the code the overview of the code.
- Link Section
-
-
All header files are included in this section which contains different functions from the libraries.
A copy of these header files is inserted into your code before compilation.
#include<stdio.h>
#include<stdio.h> is a pre-processor command, which tells a C compiler to include stdio.h file
before going to actual compilation.
- Definition Section
-
- Includes pre-processor directive, which contains symbolic constants.
E.g.: #define allows us to use constants in our code. It replaces all the constants with its
value in the code.
- Global Declaration Section
-
- main() Function
-
-
For every C program, the execution starts from the main() function. It is mandatory to include a
main() function in every C program.
Each main function contains 2 parts. A declaration part and an Execution part. The declaration part
is the part where all the variables are declared. The execution part begins with the curly brackets
and ends with the curly close bracket. Both the declaration and execution part are inside the curly braces.
void main()
{
int a, b, c;
printf(“\n Enter three number”);
scanf(“%d%d%d”, &a, &b, &c);
printf(“\nSum = %d”, callsum(a, b, c));
}
|